In this post, we will learn about the bubble sort algorithm. The bubble sort algorithm is one of the important sorting algorithms
It will compare two adjacent elements in an array and swap the right value to the left if it is lesser than left
Bubble Sort
Time Complexity: O(n^2)
Values | 10 | 12 | 4 | 6 | 2 |
Position | 0 | 1 | 2 | 3 | 4 |
Step 1 Iterate the given array using for loop Step 2 Add one more iteration within the for loop again Step 3 compare the first element with second element Step 4 If first element greater than second element swap the two elements Step 5 Compare the second element with third element Step 6 If Second element is greater than third element, then swap the two elements Step 7 Continue until nth element
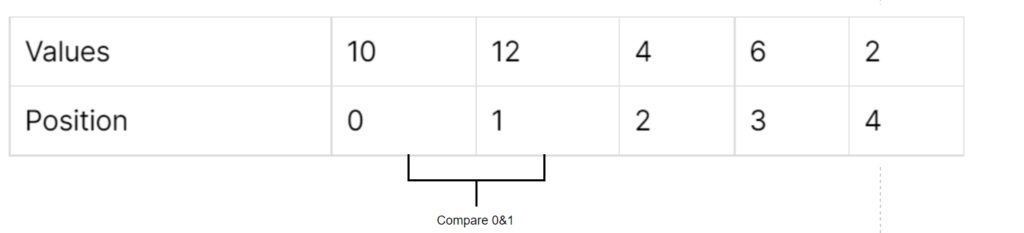
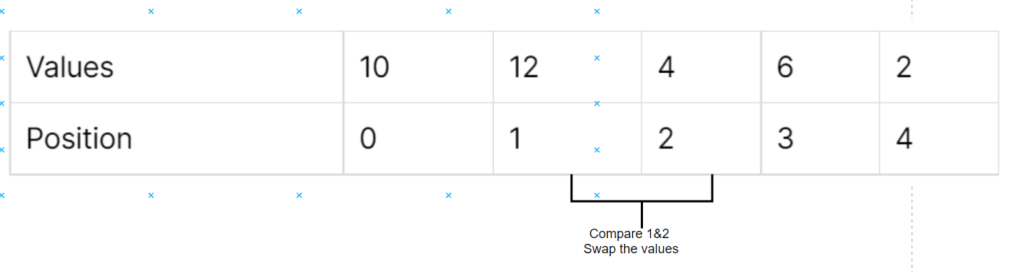
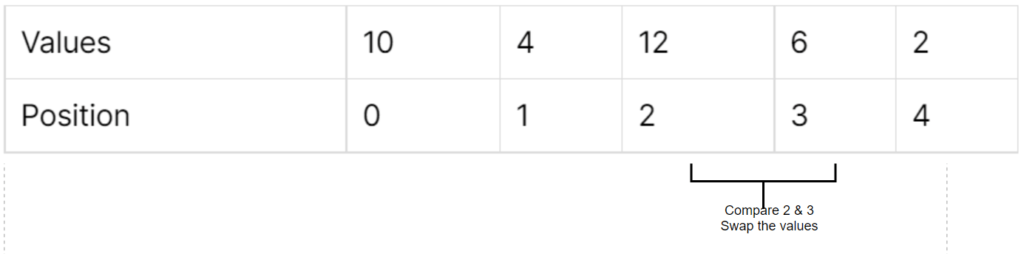
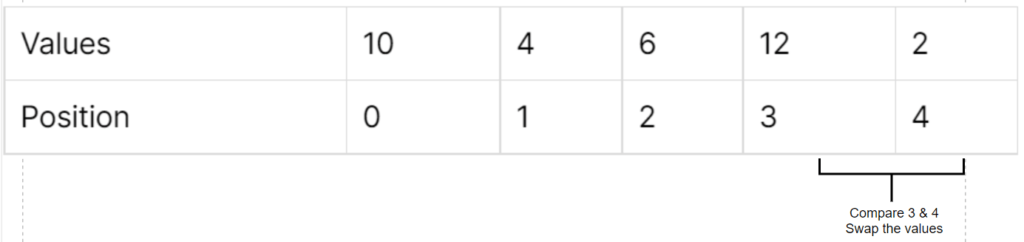

Java Implementation
public class BubbleSort {
private void sortArray(int arr[]) {
for (int i = 0; i < arr.length; i++) {
boolean isSwap = false;
for (int j = 0; j < arr.length - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
int temp = arr[j + 1];
arr[j + 1] = arr[j];
arr[j] = temp;
isSwap = true;
}
}
if (!isSwap) {
break;
}
}
}
public static void main(String[] args) {
int arr[] = { 10, 12, 4, 6, 2 };
System.out.println("Array Before sorting");
for (int i = 0; i < arr.length; i++) {
System.out.print(arr[i] + " ");
}
BubbleSort bubbleSort = new BubbleSort();
bubbleSort.sortArray(arr);
System.out.println("\nArray After Sorting");
for (int i = 0; i < arr.length; i++) {
System.out.print(arr[i] + " ");
}
}
}
Output
Array Before sorting
10 12 4 6 2
Array After Sorting
2 4 6 10 12