In this tutorial, we will learn about read excel file in python using the pandas library.
In this example, we are using the pandas library to read an excel file.
Pandas is an open-source library that has a lot of features inbuilt. Here we are using it for reading the excel file.
For More refer to official docs of the pandas
https://pandas.pydata.org/
For our exercise, I am going to use the below excel file format.
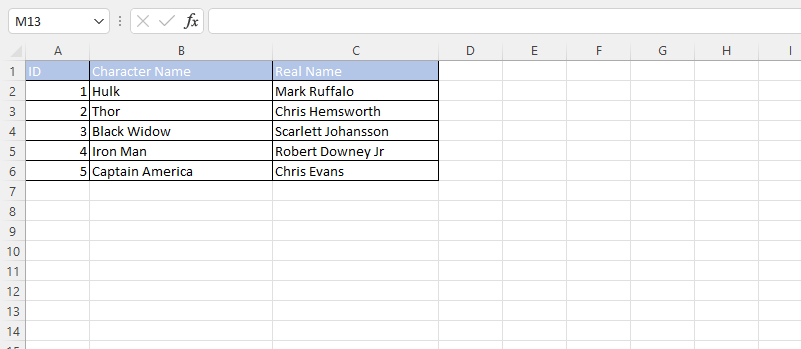
Pandas Installtion
pip install pandas
Read excel example
# Using pandas library
import pandas as panda
class ReadExcel:
# Main method
if __name__ == "__main__":
# file path of xlsx file
file_path = "D://data/Avengers.xlsx"
# reading the excel file
excel = panda.read_excel(file_path)
print(excel)
Output
ID Character Name Real Name
0 1 Hulk Mark Ruffalo
1 2 Thor Chris Hemsworth
2 3 Black Widow Scarlett Johansson
3 4 Iron Man Robert Downey Jr
4 5 Captain America Chris Evans
References
https://beginnersbug.com/create-dataframe-in-python-using-pandas/