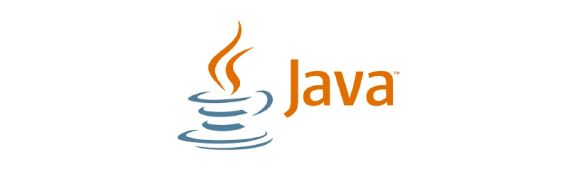
In this tutorial we will learn about Exception Handling in java
What is Exception
Any Error occurred while compiling or run time is called exception
What is exception handling
Grace fully handling that exception in our application is called exception handling
Why we need exception handling
The users should not get affected for our code bug or our environment issue,so we can handle it while developing itself
How to handle a exception
Java has strong exception handling mechanism, It has so many pre defined exception class and also it is allowing us to create our own custom exception class
How to achieve it in java
Using try, catch,throws,throw keywords we can handle exception in java
Example
public class ExceptionHandling {
public static void main(String[] args) {
try {
System.out.println(5 / 0);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Output
java.lang.ArithmeticException: / by zero
at com.geeks.overloading.ExceptionHandling.main(ExceptionHandling.java:7)
In the above code we intentionally divide a number by 0 which thrown an arithmetic exception which is caught by catch block
Finally keyword
finally is a keyword which will execute as a last part of execution, even any exception occurred also the finally method will execute
Example
public class ExceptionHandling {
public static void main(String[] args) {
try {
System.out.println(5 / 0);
} catch (Exception e) {
e.printStackTrace();
} finally {
System.out.println("The Program will exit now ");
}
}
}
Output
java.lang.ArithmeticException: / by zero
at com.geeks.overloading.ExceptionHandling.main(ExceptionHandling.java:7)
The Program will exit now
Reference
https://github.com/rkumar9090/BeginnersBug/blob/master/BegineersBug/src/com/geeks/example/ExceptionHandling.java