In this post, we will see ag grid angular examples. ag grid is one of the most commonly used grid in modern web applications. It is easy to integrate with java script, angular. react ,vue.js.
It is available in both community & enterprise edition
https://www.ag-grid.com/documentation/
In this post we will learn to integrate the ag grid with angular. We are consider that you have a angular project already
Install ag grid in angular project
Run below commands to install ag grid in the angular project, Below will install the ag grid community version to your application
npm install --save ag-grid-community ag-grid-angular
npm install
app.module.ts
Add ag-grid module in app.module.ts as like below
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { AppComponent } from './app.component';
import { AgGridModule } from 'ag-grid-angular';
@NgModule({
declarations: [AppComponent],
imports: [
BrowserModule,
AgGridModule.withComponents([])
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule {}
Ag-grid Styling
ag-grid is coming with multiple styling and themes you have to add below code in the src/style.css file
@import "../node_modules/ag-grid-community/dist/styles/ag-grid.css";
@import "../node_modules/ag-grid-community/dist/styles/ag-theme-alpine.css";
Below are the providing themes in ag grid
- ag-theme-alpine
- @import “../node_modules/ag-grid-community/dist/styles/ag-theme-alpine.css”;
- ag-theme-alpine-dark
- @import “../node_modules/ag-grid-community/dist/styles/ag-theme-alpine-dark.css”;
- ag-theme-balham
- @import “../node_modules/ag-grid-community/dist/styles/ag-theme-balham.css”;
- ag-theme-balham-dark
- @import “../node_modules/ag-grid-community/dist/styles/ag-theme-balham-dark.css”
- ag-theme-material
- @import “../node_modules/ag-grid-community/dist/styles/ag-theme-material.css”
Adding grid in angular
We have to add column definition and row data in the component.ts file, Below is the snippet of app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'ag-custom';
columnDefs = [
{ headerName: "Make", field: "make" ,resizable: true,filter: true },
{ headerName: "Model", field: "model",resizable: true ,filter: true },
{ headerName: "Price", field: "price",resizable: true ,filter: true }
];
rowData = [
{ make: "Toyota", model: "Celica", price: 35000 },
{ make: "Ford", model: "Mondeo", price: 32000 },
{ make: "Porsche", model: "Boxter", price: 72000 }
];
}
In the app.component.html file add the below snippet
<div>
<ag-grid-angular style="width: 100%; height: 500px;" class="ag-theme-alpine" [rowData]="rowData"
[columnDefs]="columnDefs">
</ag-grid-angular>
</div>
Output
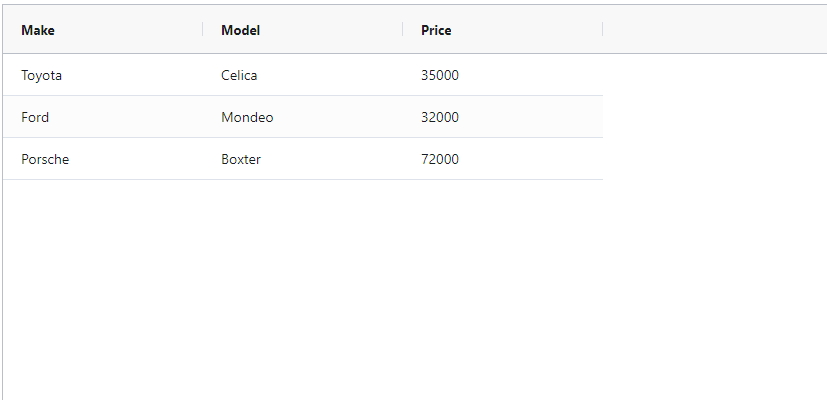