lazy loading in angular is one of the most important concept. You have to understand this while designing an enterprise level angular app
What is lazy loading ?
In single page application we will load all the components and required java script and css files in single shot
But this will take more time when we have more components and more dependencies.
In order to avoid this angular has cool feature which is called lazy loading. which will load required module on need basis
ngModule
ngModule will allow us to group the things together, Module is great way to organize an application
Every angular application will have one base module which is called appModule
@NgModule
decorator will make class as module. which will come with declarations,providers,imports. Below is the sample module
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { LazyRoutingModule } from './lazy-routing.module';
import { LazyComponent } from 'src/app/lazy/lazy/lazy.component';
import { MaterialModule } from '../material/material.module';
import { MatTabsModule } from '@angular/material/tabs';
import {MatCardModule} from '@angular/material/card';
import { SampleComponent } from 'src/app/lazy/sample/sample/sample.component';
import {MatButtonModule} from '@angular/material/button';
@NgModule({
declarations: [LazyComponent,SampleComponent],
imports: [
CommonModule,
LazyRoutingModule,
MaterialModule,
]
})
export class LazyModule { }
Lazy Loading Steps
- Create new angular project
- ng new lazy-example –routing
- Create new component(HomeComponent)
- ng new component home
- Create new Component (LazyComponent)
- ng new component lazy
- Create new module(LazyModule)
- ng new module lazy –routing
Below is the folder structure of our application
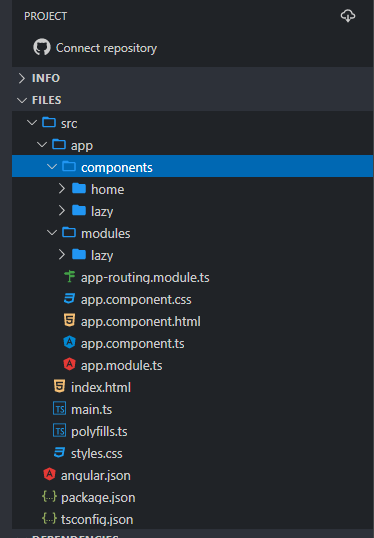
We have two components.& two Modules
- AppModule is the base module used while application launch
- LazyModule is the lazy module used while user clicks the lazy url
- HomeComponent will get initialized while application launch via appModule
- LazyComponent will get initialized while clicking the lazy routing url via LazyModule
Declare the HomeComponent in AppModule as like below
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
import { AppComponent } from './app.component';
import { BrowserAnimationsModule } from '@angular/platform-browser/animations';
import { AppRoutingModule } from './app-routing.module';
import { HomeComponent } from './components/home/home.component';
@NgModule({
imports: [
BrowserModule,
FormsModule,
AppRoutingModule,
BrowserAnimationsModule
],
declarations: [AppComponent, HomeComponent],
bootstrap: [AppComponent]
})
export class AppModule {}
Declare the LazyComponent in LazyModule as like below
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { LazyRoutingModule } from './lazy-routing.module';
import { MatTabsModule } from '@angular/material/tabs';
import { LazyComponent } from '../../components/lazy/lazy.component';
@NgModule({
imports: [CommonModule, LazyRoutingModule, MatTabsModule],
declarations: [LazyComponent]
})
export class LazyModule {}
The important point is routing. We have to mention that load children (LazyModule) in app-routing.module.ts
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { RouterModule, Routes } from '@angular/router';
import { LazyModule } from './modules/lazy/lazy.module';
import { HomeComponent } from './components/home/home.component';
const routes: Routes = [
{ path: 'home', component: HomeComponent },
{ path: 'lazy', loadChildren: () => LazyModule }
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule],
declarations: []
})
export class AppRoutingModule {}
As like app-routing we have to mention the home path in lazy-routing.module.ts file as like below
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
import { LazyComponent } from '../../components/lazy/lazy.component';
const routes: Routes = [{ path: '', component: LazyComponent }];
@NgModule({
imports: [RouterModule.forChild(routes)],
declarations: []
})
export class LazyRoutingModule {}
That’s it you can see the output as like below
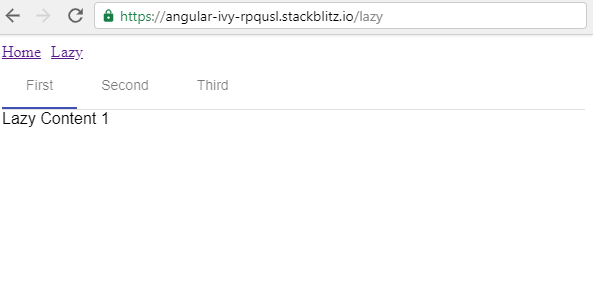
StackBlitz URL:
https://stackblitz.com/edit/angular-ivy-rpqusl?file=src/app/app.component.ts